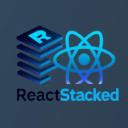
How To Boost React Native Accessibility In 2025
Enhance react native accessibility in 2025 with tools and practices for inclusive apps.
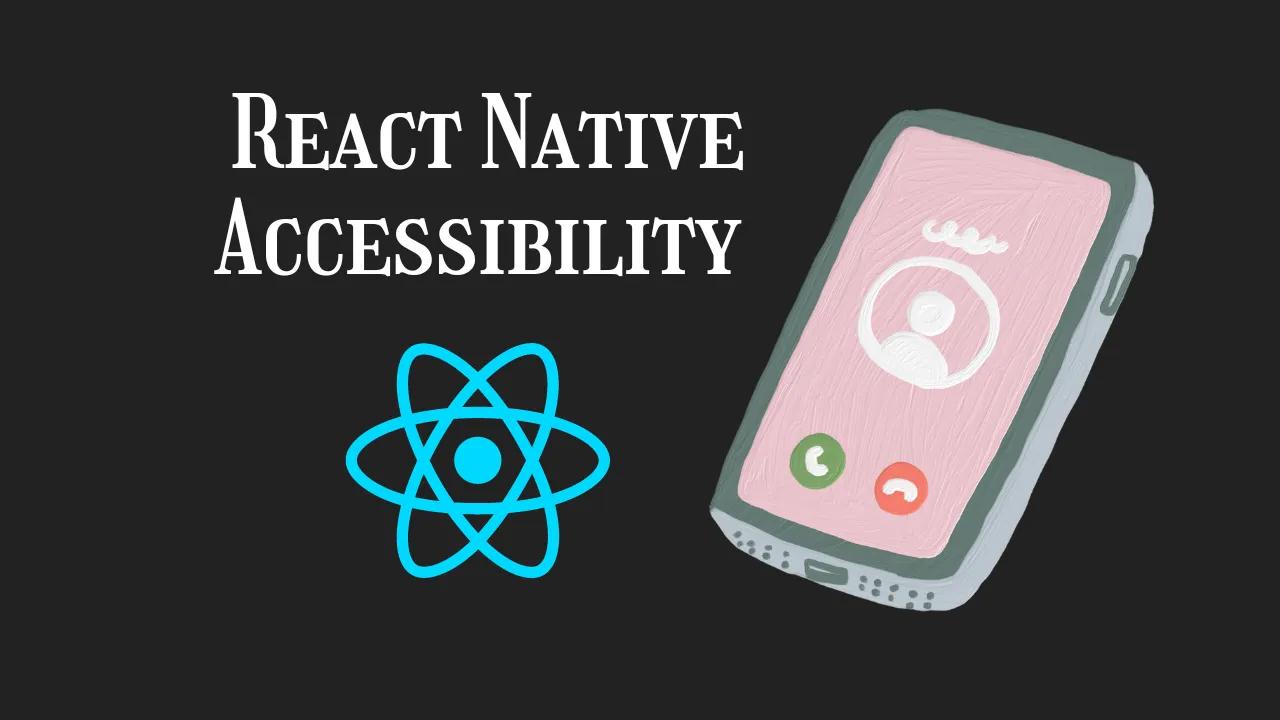
As of April 9, 2025, accessibility is a trending topic in mobile development, driven by stricter regulations and user demand for inclusion. React Native offers robust tools to build accessible apps for all. This article explores how to boost accessibility in React Native, covering setup, UI enhancements, screen reader support, dynamic adjustments, testing, performance considerations, and deployment strategies to create inclusive apps in 2025.
Set Up an Accessible Foundation
Start with a fresh project: npx react-native init AccessApp --version latest
. Leverage the New Architecture, standard in 2025, for better rendering control. Install TypeScript (npm install typescript @types/react @types/node
) and set tsconfig.json
with "strict": true
for type-safe accessibility props.
For Expo, use npx create-expo-app AccessApp --template bare-minimum
. Add react-native-accessibility-engine
(npm install react-native-accessibility-engine
) to audit components early. This setup aligns with 2025’s trend toward accessibility-first development.
Enhance UI for Accessibility
Accessible UI starts with clear components. Add ARIA-like props to elements:
import { TouchableOpacity, Text } from 'react-native';
export default function Button({ onPress, label }) {
return (
<TouchableOpacity
accessible={true}
accessibilityLabel={label}
accessibilityRole="button"
onPress={onPress}
>
<Text>{label}</Text>
</TouchableOpacity>
);
}
Use accessibilityLabel
for screen readers and accessibilityRole
to define purpose. In 2025, trends favor semantic markup, ensuring VoiceOver (iOS) and TalkBack (Android) interpret UIs correctly.
Optimize for Screen Readers
Screen readers need focus management. Group related elements with accessibilityElementsHidden
:
import { View, Text } from 'react-native';
export default function Card({ title, description }) {
return (
<View accessible={true} accessibilityLabel={`${title}, ${description}`}>
<Text>{title}</Text>
<Text accessibilityElementsHidden={true}>{description}</Text>
</View>
);
}
Set importantForAccessibility="no"
on decorative items to reduce clutter. In 2025, live regions trend for dynamic updates: accessibilityLiveRegion="polite"
announces changes like form errors without interrupting users.
Support Dynamic Adjustments
Users adjust settings like font size. Handle dynamic type with Text
:
import { Text, PixelRatio } from 'react-native';
export default function ScalableText({ children }) {
const scale = PixelRatio.getFontScale();
return (
<Text style={{ fontSize: 16 * scale }} allowFontScaling={true}>
{children}
</Text>
);
}
Respect motion preferences with useReducedMotion
from react-native-reanimated
:
import { useReducedMotion } from 'react-native-reanimated';
export default function AnimatedView({ children }) {
const reducedMotion = useReducedMotion();
return reducedMotion ? <View>{children}</View> : <Animated.View>{children}</Animated.View>;
}
In 2025, this trend ensures apps adapt to user needs, meeting WCAG 2.2 standards.
Test Accessibility Thoroughly
Testing validates inclusion. Use Jest (npm install --save-dev jest
) and React Native Testing Library (@testing-library/react-native
) for units:
import { render } from '@testing-library/react-native';
test('Button has accessible label', () => {
const { getByA11yLabel } = render(<Button label="Submit" onPress={() => {}} />);
expect(getByA11yLabel('Submit')).toBeTruthy();
});
For e2e, Detox (npm install --save-dev detox
) checks real devices: await element(by.label('Submit')).tap()
. Manually test with VoiceOver and TalkBack on emulators, toggling settings. In 2025, tools like expo-accessibility
trend for automated audits.
Optimize Performance with Accessibility
Accessibility shouldn’t slow apps. Use the New Architecture’s Fabric for efficient rendering, enabled in app.json
with "newArchEnabled": true
. Avoid heavy re-renders with React.memo
:
import { memo } from 'react';
import { Text } from 'react-native';
const Label = memo(({ text }) => (
<Text accessible={true} accessibilityLabel={text}>{text}</Text>
));
Profile with Flipper’s Accessibility plugin to ensure 60 FPS, even with screen readers active. In 2025, this trend balances inclusion and speed on low-end devices.
Deploy Accessible Apps
Deployment ensures accessibility reaches users. With Expo EAS (eas build --platform all
), embed accessibility configs and update via OTA. For bare apps, use Fastlane (fastlane deploy
) with a CI/CD pipeline, testing a11y in staging.
Monitor with Sentry (npm install @sentry/react-native
), catching focus or label issues: Sentry.captureMessage('A11y error')
. In 2025, trends like App Clips (react-native-branch
) highlight accessible features instantly, optimized for all users.
Enhance with Real-World Scenarios
Accessibility shines in practice. Build a form with clear feedback:
import { TextInput, Text } from 'react-native';
import { useState } from 'react';
export default function Form() {
const [error, setError] = useState('');
return (
<View>
<TextInput
accessible={true}
accessibilityLabel="Email"
onChangeText={(text) => !text.includes('@') && setError('Invalid email')}
/>
<Text accessibilityLiveRegion="assertive">{error}</Text>
</View>
);
}
For navigation, use React Navigation with focusable screens. In 2025, accessible fitness trackers or e-commerce apps trend, ensuring all users can engage fully.
Conclusion
Boosting React Native accessibility in 2025 creates inclusive, user-friendly apps. With semantic UI, dynamic support, and rigorous testing, you can meet modern standards. Start with these practices, optimize for all, and lead in mobile inclusion!