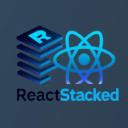
React Native Tailwind Css Installation With Nativewind
We'll install Tailwind CSS to our react native app using Nativewind to build mobile apps much more easily.
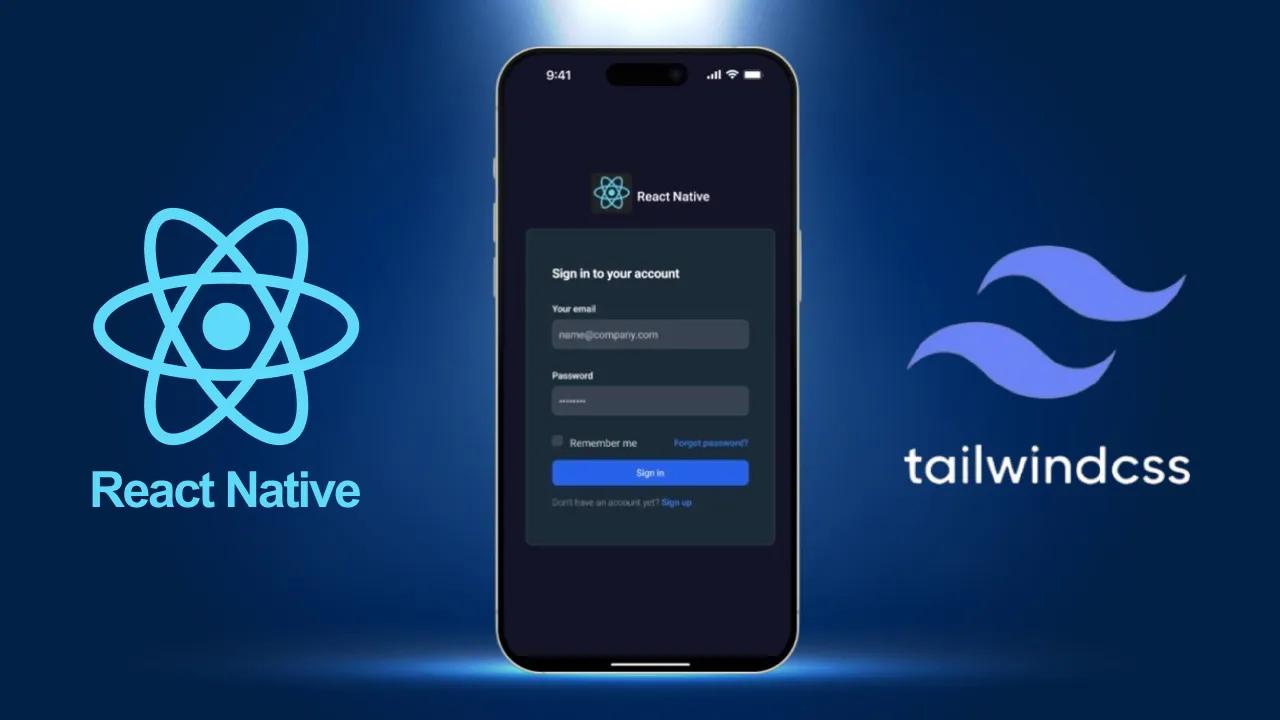
You can also watch the YouTube video:
React Native Tailwind CSS Installation with Nativewind
I'll use "yarn" as package manager but you can use whatever you want.
If you want to install and use yarn you can install with one command:
npm i -g yarn
Create a new application:
npx @react-native-community/cli@latest init RNTailwind
Once the installation done:
cd RNTailwind
Delete package-lock.json if you'll use "yarn" package manager.
Use yarn
command to generate yarn-lock.json
file:
yarn
Start the app:
yarn start
Run app on android or iOs(I'll use android):
yarn android
- Install NativeWind
You will need to install Nativewind and it's peer dependencies tailwindcss, react-native-reanimated and react-native-safe-area-context
yarn add nativewind tailwindcss react-native-reanimated react-native-safe-area-context
For Mac users run:
npx pod-install
- Setup Tailwind CSS
Run npx tailwindcss init
to create a tailwind.config.js file
Add the paths to all of your component files in your tailwind.config.js file.
/** @type {import('tailwindcss').Config} */
module.exports = {
// NOTE: Update this to include the paths to all of your component files.
content: ['./app/**/*.{js,jsx,ts,tsx}'],
presets: [require('nativewind/preset')],
theme: {
extend: {},
},
plugins: [],
};
Create a app
folder and create globals.css
CSS file and add the Tailwind directives:
/* app/globals.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
- Add the Babel preset:
// babel.config.js
module.exports = {
presets: ["module:@react-native/babel-preset", "nativewind/babel"],
};
- Modify your metro.config.js
// metro.config.js
const { getDefaultConfig } = require("@react-native/metro-config");
const { withNativeWind } = require("nativewind/metro");
const config = getDefaultConfig(__dirname);
module.exports = withNativeWind(config, { input: "./app/globals.css" });
- Move your
App.tsx(jsx)
file toapp
folder and import your CSS file
import React from "react";
import {
SafeAreaView,
ScrollView,
StatusBar,
Text,
useColorScheme,
View,
} from "react-native";
import "./globals.css";
const App = (): React.JSX.Element => {
const isDarkMode = useColorScheme() === "dark";
const backgroundStyle = "bg-neutral-300 dark:bg-slate-900 h-full";
return (
<SafeAreaView className={backgroundStyle}>
<StatusBar barStyle={isDarkMode ? "light-content" : "dark-content"} />
<ScrollView contentInsetAdjustmentBehavior="automatic">
<View>
<Text className="text-3xl font-bold text-black dark:text-white">
Hello World!
</Text>
</View>
</ScrollView>
</SafeAreaView>
);
};
export default App;
If you get an error like:
package="com.th3rdwave.safeareacontext" found in source AndroidManifest.xml: C:\Users\Victus\Downloads\RNTailwind\node_modules\react-native-safe-area-context\android\src\main\AndroidManifest.xml
Edit the AndroidManifest.xml in react-native-safe-area:
<manifest
xmlns:android="http://schemas.android.com/apk/res/android">
</manifest>
If you get an error like this:
[cxx1429] Error When Building With Cmake Using
Check this post:
React Native [cxx1429] Error When Building With Cmake Using Solution
- TypeScript (optional)
NativeWind extends the React Native types via declaration merging. The simplest method to include the types is to create a new nativewind-env.d.ts
file and add a triple-slash directive referencing the types.
Our Nativewind installation is done, now we can make a simple login screen to see how it's works.
Edit App.tsx
:
import React from "react";
import {
SafeAreaView,
ScrollView,
StatusBar,
useColorScheme,
View,
} from "react-native";
import SignIn from "./components/SignIn";
import "./globals.css";
const App = (): React.JSX.Element => {
const isDarkMode = useColorScheme() === "dark";
const backgroundStyle = "bg-gray-50 dark:bg-gray-900 h-full";
return (
<SafeAreaView className={backgroundStyle}>
<StatusBar barStyle={isDarkMode ? "light-content" : "dark-content"} />
<ScrollView contentInsetAdjustmentBehavior="automatic">
<View>
<SignIn />
</View>
</ScrollView>
</SafeAreaView>
);
};
export default App;
Create components
folder inside the app
folder and create SignIn
component:
import { Image, Text, TextInput, TouchableOpacity, View } from "react-native";
const SignIn = () => {
return (
<View className="m-4">
<View className="flex flex-col items-center justify-center px-6 py-8 h-screen">
<TouchableOpacity className="flex flex-row items-center mb-6 text-2xl font-semibold text-gray-900 dark:text-white">
<Image
className="w-16 h-16 mr-2"
source={{
uri: "https://reactnative.dev/img/tiny_logo.png",
}}
alt="Logo"
/>
<Text className="mt-2 text-xl font-medium text-black dark:text-white">
React Native
</Text>
</TouchableOpacity>
<View className="w-full bg-white py-8 px-4 rounded-lg shadow dark:border dark:bg-gray-800 dark:border-gray-700">
<View className="p-6">
<Text className="text-xl font-bold leading-tight tracking-tight text-gray-900 dark:text-white">
Sign in to your account
</Text>
<View className="mt-8">
<View>
<Text className="block mb-2 text-sm font-medium text-gray-900 dark:text-white">
Your email
</Text>
<TextInput
className="bg-gray-50 border border-gray-300 text-gray-900 rounded-lg block w-full p-2.5 dark:bg-gray-700 dark:border-gray-600 dark:placeholder-gray-400 dark:text-white"
placeholder="[email protected]"
/>
</View>
<View className="mt-8">
<Text className="block mb-2 text-sm font-medium text-gray-900 dark:text-white">
Password
</Text>
<TextInput
placeholder="••••••••"
className="bg-gray-50 border border-gray-300 text-gray-900 rounded-lg block w-full p-2.5 dark:bg-gray-700 dark:border-gray-600 dark:placeholder-gray-400 dark:text-white"
/>
</View>
<View className="flex flex-row items-center justify-between mt-8">
<View className="flex flex-row items-start">
<View className="flex items-center">
<TouchableOpacity className="w-4 h-4 border border-gray-300 rounded bg-gray-50 dark:bg-gray-700 dark:border-gray-600" />
</View>
<View className="ml-3 text-sm">
<Text className="text-gray-500 dark:text-gray-300">
Remember me
</Text>
</View>
</View>
<Text className="text-sm font-medium text-blue-600 dark:text-blue-500">
Forgot password?
</Text>
</View>
<TouchableOpacity className="mt-4 w-full bg-blue-600 font-medium rounded-lg px-5 py-2.5">
<Text className="text-white text-sm text-center">Sign in</Text>
</TouchableOpacity>
<Text className="mt-4 text-sm font-light text-gray-500 dark:text-gray-400">
Don't have an account yet?{" "}
<Text className="font-medium text-blue-600 dark:text-blue-500">
Sign up
</Text>
</Text>
</View>
</View>
</View>
</View>
</View>
);
};
export default SignIn;
Thank you for reading my blog post I hope you found it useful.